Converting data type to numeric is important while analyzing the data in R. In this tutorial, we will learn three ways of converting the colums of data frame to numeric. Find out how to convert all columns of data frame to numeric in R.
In this tutorial, we learn three ways of converting all data frame columns to numeric in R. Firstly, we go over dplyr package to convert the columns to numeric in data frame. Secondly, we work on sapply() function to convert all columns to numeric in R data frame. At last, we learn how to convert all columns of data frame to numeric in R using apply() function.
Let’s construct a data frame including the variables with different classes as an example data frame.
a <- c(1,3,5,-4)
b <- c("5","3","1","4")
c <- c(3L,-2L,4L,7L)
data <- data.frame(a,b,c)
data
## a b c
## 1 1 5 3
## 2 3 3 -2
## 3 5 1 4
## 4 -4 4 7
sapply(data, class)
## a b c
## "numeric" "character" "integer"
Check Out: How to Find Class of Each Column in R Data Frame
1) How to Convert All Data Frame Columns to Numeric in R Using dplyr Package
In this part, we use mutate_at() function available in dplyr package to convert the columns to numeric in data frame.
library(dplyr)
data2 <- data %>% mutate_at(1:3, as.numeric)
data2
## a b c
## 1 1 5 3
## 2 3 3 -2
## 3 5 1 4
## 4 -4 4 7
sapply(data2, class)
## a b c
## "numeric" "numeric" "numeric"
Also Check: How to Clean Data in R
2) How to Convert All Data Frame Columns to Numeric in R Using sapply() Function
In this section, we learn sapply() function to change the classes of all data frame columns to numeric in R. When we use sapply() function, the class of data frame becomes matrix or array. Therefore, we need to convert the class of data to data frame with as.data.frame() function.
data2 <- sapply(data, as.numeric)
data2 <- as.data.frame(data2)
data2
## a b c
## 1 1 5 3
## 2 3 3 -2
## 3 5 1 4
## 4 -4 4 7
sapply(data2, class)
## a b c
## "numeric" "numeric" "numeric"
Also Check: How to Remove Outliers from Data in R
3) How to Convert All Data Frame Columns to Numeric in R Using apply() Function
In this part, we work on apply() function to change the classes of all data frame columns to numeric in R. When we use apply() function, the class of data frame becomes matrix or array. Therefore, we need to convert the class of data to data frame with as.data.frame() function.
data2 <- apply(data, 2, function(x) as.numeric(x))
data2 <- as.data.frame(data2)
data2
## a b c
## 1 1 5 3
## 2 3 3 -2
## 3 5 1 4
## 4 -4 4 7
sapply(data2, class)
## a b c
## "numeric" "numeric" "numeric"
The application of the codes is available in our youtube channel below.
Don’t forget to check: How to Sort a Data Frame by Single and Multiple Columns in R
References
Wickham, H., Francois, R., Henry, L., Muller, K. (2022). dplyr: A Grammar of Data Manipulation. R package version 1.0.10.
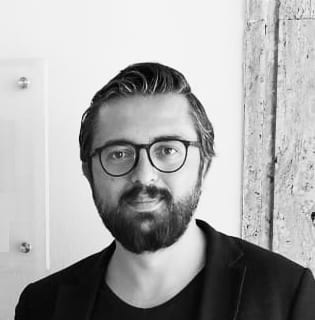
0 Comments
1 Pingback